RESAS APIを使ってみる(2)
投稿日: 2017年01月23日 更新日: 2017年07月22日
前回に引き続きRESAS APIの使い方です。
本記事では実際にサーバサイドのWebアプリケーションからRESAS APIを使ってデータを取得してみます。
※現状、RESAS APIのAPIキーは非公開を前提としているのでJavaScriptなどのクライアントサイドプログラムでの利用は推奨されません。
サーバサイドのプログラム言語にはPHPを使い、環境にはHerokuを使います。
1. 今回作成するWebアプリケーション
都道府県と市区町村を選択し、自然人口の増減をグラフ表示するWebアプリケーションを作ってみましょう。
2. Herokuの環境を準備する
SIGN UP FOR FREEからアカウントの登録をします。
次にHeroku CLIをダウンロードしインストールします。
https://devcenter.heroku.com/articles/heroku-cli
※私の32bit Windows7環境下ではGitがインストールできなかったので、Git for Windowsを別途インストールしました。
https://git-for-windows.github.io/
3. Webアプリケーションを作ってみる
まずはHerokuにログインします。
$ heroku login
空のPHPアプリケーションを作成します。
$ heroku create <APP_NAME>
$ mkdir <APP_NAME>
$ cd <APP_NAME>
$ touch composer.json
$ touch index.php
$ git init
$ git add .
$ heroku git:remote -a <APP_NAME>
$ git commit -m “first commit”
$ git push heroku master
$ heroku open
ブラウザでhttps://
index.phpを以下のように書き換えます。
今回のプログラムでは都道府県の選択画面を実装します。
<?php
// エラーハンドリングやセキュリティ対策はしていません。実コードでは気をつけましょう。
$base_url = 'https://opendata.resas-portal.go.jp/';
$request_url = $base_url . 'api/v1/prefectures';
$headers = ['X-API-KEY: <API-KEY>'];
$curl = curl_init();
curl_setopt($curl, CURLOPT_HTTPHEADER, $headers);
curl_setopt($curl, CURLOPT_RETURNTRANSFER, TRUE);
// 都道府県を取得
curl_setopt($curl, CURLOPT_URL, $request_url);
$response = curl_exec($curl);
// 都道府県一覧のJSONを配列に格納
$prefectures = json_decode($response, true)['result'];
?>
<html>
<head>
<title>都道府県一覧</title>
</head>
<body>
<form action="cities.php" method="get">
<select name="prefCode">
<?php foreach($prefectures as $pref): ?>
<option value="<?=htmlspecialchars($pref['prefCode']) ?>"><?= htmlspecialchars($pref['prefName']);?> </option>
<?php endforeach; ?>
</select>
<button type="submit">選択</button>
</form>
<?php ?>
</body>
</html>
書き換えたらDEPLOYします。
$ git commit -am “modified index.php”
$ git push heroku master
$ heroku open
次に、市区町村の選択画面をcities.phpとして実装します。
<?php
// エラーハンドリングやセキュリティ対策はしていません。実コードでは気をつけましょう。
$base_url = 'https://opendata.resas-portal.go.jp/';
$request_url = $base_url . 'api/v1/cities';
$headers = ['X-API-KEY: <API-KEY>'];
$curl = curl_init();
curl_setopt($curl, CURLOPT_HTTPHEADER, $headers);
curl_setopt($curl, CURLOPT_RETURNTRANSFER, TRUE);
// 市区町村を取得
curl_setopt($curl, CURLOPT_URL, $request_url . '?prefCode=' . rawurlencode($_GET['prefCode']));
$response = curl_exec($curl);
// 市区町村一覧のJSONを配列に格納
$cities = json_decode($response, true)['result'];
// print_r($cities);
?>
<html>
<head>
<title>市区町村一覧</title>
</head>
<body>
<p><a href="index.php">都道府県選択に戻る</a></p>
<form action="population.php" method="get">
<input type="hidden" name="prefCode" value="<?=htmlspecialchars($_GET[prefCode]); ?>" />
<select name="cityCode">
<?php foreach($cities as $city): ?>
<option value="<?=htmlspecialchars($city['cityCode']) ?>"><?= htmlspecialchars($city['cityName']);?> </option>
<?php endforeach; ?>
</select>
<button type="submit">選択</button>
</form>
<?php ?>
</body>
</html>
再びDEPLOYします。
$ git add .
$ git commit -m “add cities.php”
$ git push heroku master
$ heroku open
最後に、人口の自然増減をグラフ上にプロットするためのpopulation.phpを実装します。グラフの表示にはGoogle Chartsを使いました。
https://developers.google.com/chart/
<?php
// エラーハンドリングやセキュリティ対策はしていません。実コードでは気をつけましょう。
$base_url = 'https://opendata.resas-portal.go.jp/';
$request_url = $base_url . 'api/v1/population/nature';
$headers = ['X-API-KEY: <API-KEY>'];
$curl = curl_init();
curl_setopt($curl, CURLOPT_HTTPHEADER, $headers);
curl_setopt($curl, CURLOPT_RETURNTRANSFER, TRUE);
// 市区町村を取得
curl_setopt($curl, CURLOPT_URL, $request_url . '?prefCode=' . rawurlencode($_GET['prefCode']) . '&cityCode=' . rawurlencode($_GET['cityCode']));
$response = curl_exec($curl);
// 人口増減のJSONを配列に格納
$population = json_decode($response, true);
?>
<html>
<head>
<title>自然人口増減(男性のみ)</title>
<script type="text/javascript" src="https://www.gstatic.com/charts/loader.js"></script>
<script type="text/javascript">
google.charts.load('current', {'packages':['bar']});
google.charts.setOnLoadCallback(drawChart);
function drawChart() {
var arrData = [['Year', 'population'],
<?php foreach($population['result']['bar']['mandata'] as $mandata): ?>
['<?=$mandata['year']?>', <?=$mandata['value'];?>],
<?php endforeach; ?>];
var data = google.visualization.arrayToDataTable(arrData);
var options = {
bars: 'vertical',
hAxis: {
title: 'Man Population',
minValue: 0,
}
};
var chart = new google.charts.Bar(document.getElementById('barchart'));
chart.draw(data, options);
}
</script>
</head>
<body>
<p><a href="cities.php?prefCode=<?=rawurlencode($_GET['prefCode']); ?>">市区町村選択に戻る</a></p>
<div id="barchart" style="width: 900px; height: 500px;"></div>
</body>
</html>
DEPLOY方法はさきほどと同様です。
$ git add .
$ git commit -m “add population.php”
$ git push heroku master
$ heroku open
4. 最後に
以上でWebアプリケーションは一旦完成です。
表示されないなどの不具合があった再には以下のコマンドでログを確認することができます。
Heroku logs
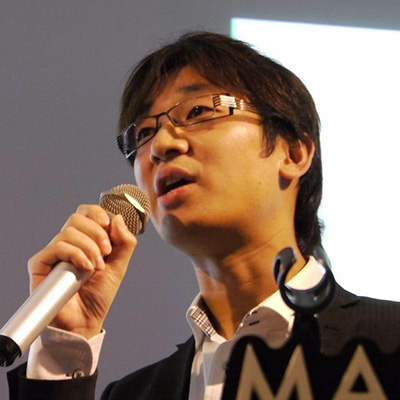
名前:宮内 はじめ
Code for Nagoya名誉代表
E2D3名古屋支部長
プログラマーです。GISやデータビズが好きです。このサイトは宮内の個人的なメモです。